Key Highlights
- This blog post provides a curated set of Python interview coding questions to help you prepare.
- It covers fundamental and advanced topics, from basic data structures to complex algorithms.
- Each question is presented with a detailed explanation and code examples for clarity.
- Familiarity with these questions will enhance your problem-solving skills.
- Prepare to impress interviewers and demonstrate your Python programming expertise!
Introduction
In software development, it is important to master a flexible programming language like Python. If you are getting ready for your next Python interview, it is a good idea to know some common questions that might come up. This blog post lists Python programming interview questions that will test your understanding of key concepts and your problem-solving skills.
Python’s popularity has soared in recent years, establishing itself as one of the most widely used programming languages. According to GitHub, Python usage saw a 22.5% year-over-year increase in 2022. Its readability, simplicity, and versatility make it an ideal choice for various applications. As of 2024, Python ranks as the most popular programming language with a 28.11% market share. This widespread adoption has led to a booming job market, with over 11,000 Python-related job postings on Glassdoor and around 14,000 on Indeed. The demand for Python skills is reflected in competitive salaries, with Python developers earning an average of $111,781 per year. As organizations across industries embrace digital transformation, Python has become the go-to language for developers, driving the demand for skilled Python professionals.
Top 10 Python Interview Coding Questions You Should Prepare
Technical interviews can be stressful, especially if you are not ready. For new Python developers, showing off your coding skills is very important. This part gives you a list of ten key Python coding questions that you often see in interviews.
By learning these questions and practicing your answers, you will feel more sure of yourself. This will help you improve your problem-solving skills and might boost your chances of getting that dream job!
Question 1: Implementing a Basic Python Calculator for Arithmetic Operations
As a Python developer, you may face questions that test your skill in making simple applications. A calculator is one example that shows how well you can handle math tasks in Python.
Python is an interpreted language, which means you can run code right away. This feature makes it great for testing and trying out ideas quickly. You can write a simple calculator using conditional statements like if, elif, and else to check what the user wants to do.
This question checks your basic knowledge of input and output, operators, and how control flow works in Python.
Question 2: Writing a Python Function to Find the Factorial of a Number
The factorial of a number is what you get when you multiply all positive whole numbers that are less than or equal to that number. You often see this problem in Python interviews because it mixes math with coding skills.
To solve the factorial problem, many people write a Python function. This creates a block of code that can be used to find the factorial of any number. In the function, a local variable is usually used to keep track of the results while you calculate.
This question tests how well you understand functions and where variables are used. It also checks how you can turn a math idea into code.
Question 3: Developing a Python Script to Merge Two Sorted Lists
Merging sorted lists is an important task in computer science. You may come across this question in a Python interview. It focuses on your skills in thinking through algorithms and managing data.
You can write a Python script to make a new list that combines elements from two input lists that are already sorted. You can use list comprehension, which is a handy feature of Python, to create the merged list simply.
This question checks how well you can work with lists. It looks at your ability to go through elements and add them to a new list based on certain conditions, all while keeping the order sorted.
Question 4: Creating a Python Program to Reverse a String Without Using Built-in Functions
Reversing a string is a well-known programming task. It shows how well you understand string manipulation. In Python, strings cannot change. This means you cannot just change them directly. To reverse a string without built-in functions, you need to make a new string.
You can solve this problem by using loops and string addition. You take the original string and go through it backwards. As you go, you add each character to the start of the new string.
This task shows you can work with strings, understand that they cannot change, and come up with ways to solve the problem.
Question 5: Solving the Fibonacci Sequence Using Recursion in Python
The Fibonacci sequence is a list of numbers. Each number is the total of the two numbers before it. This is a popular question in interviews. It helps check your grasp of recursive algorithms.
In Python, you can write the Fibonacci sequence using recursion. A recursive function uses itself in its process to tackle smaller parts of a problem. It is very important to set a base case in a recursive function. This helps you avoid running in circles with endless loops.
Learning recursion and using it for problems like the Fibonacci sequence is a great skill for Python developers. This skill is useful for tasks like tree traversals and creating fractals.
Question 6: Crafting a Python Function to Check Prime Numbers in a List
Identifying prime numbers in a list is a common task in interviews. This task mixes math knowledge with Python coding skills. Prime numbers can only be divided by one and themselves. They play an important role in many algorithms.
The Python code for this task usually means creating a function. This function loops through the list and checks if each number is prime. It is key to know the properties of prime numbers for making this code better. For example, first, you may want to create an empty list to store the prime numbers. You only need to check numbers up to the square root of the number you are testing.
Using loops and conditions in your function shows your understanding of Python’s way of writing and its logic.
Question 7: Designing a Python Script to Convert Temperature from Celsius to Fahrenheit and Vice Versa
Temperature conversion from Celsius to Fahrenheit and the other way around is a common problem that tests basic programming skills. This problem checks if you can get user input, do calculations, and show results.
To create a Python script for this task, you start by taking the user’s input for the temperature and the scale (Celsius or Fahrenheit). Depending on the scale provided, you will use the correct formula to convert the temperature to the other scale.
The script shows that you know about data types, especially when you take numbers from the user and change them between units. It also shows that you can set up a program that runs steps in a specific order, which highlights how you understand program flow.
Question 8: Building a Python Program for Binary Search on a Sorted List
To use binary search in Python for a sorted list, follow these steps. First, create a function that takes the sorted list and the target value. Use the divide-and-conquer method by setting the low and high indices at the start. Next, find the middle index and compare the target value to the element at this middle index. Depending on the comparison, change the low or high indices. Keep doing this until you find the target value or the search range becomes empty. This method makes searching much quicker, reducing the time needed to logarithmic levels.
Question 9: Implementing a Python Code to Count the Occurrence of a Specific Character in a String
Counting how many times a specific character appears in a string is an important task in string handling. You might see versions of this question in job interviews. It helps to check how well you can go through and understand strings.
In Python, this task mainly uses loops to go through the string representation. Each time a character is found, it is compared to the target character. If they are the same, a counter goes up by one.
This question tests your knowledge of how to work with strings in Python. It focuses on your ability to move through strings and use variables to count or keep track of results.
Question 10: Writing a Python Program to Sort Words in Alphabetic Order
Sorting is an important process in computer science. During a Python interview, you will often face questions about sorting algorithms. Even though Python has built-in functions for sorting, it is essential to understand how these algorithms work.
You may need to write a Python program. For example, you could be asked to sort a list of words in alphabetical order. This helps show what you know about sorting algorithms. You can use different methods like Bubble Sort, Insertion Sort, or more advanced techniques like Merge Sort or Quick Sort.
Doing this will not only show your understanding of different sorting methods but also your ability to solve problems. You will need to choose the best algorithm based on the size of the list and how efficient you want it to be.
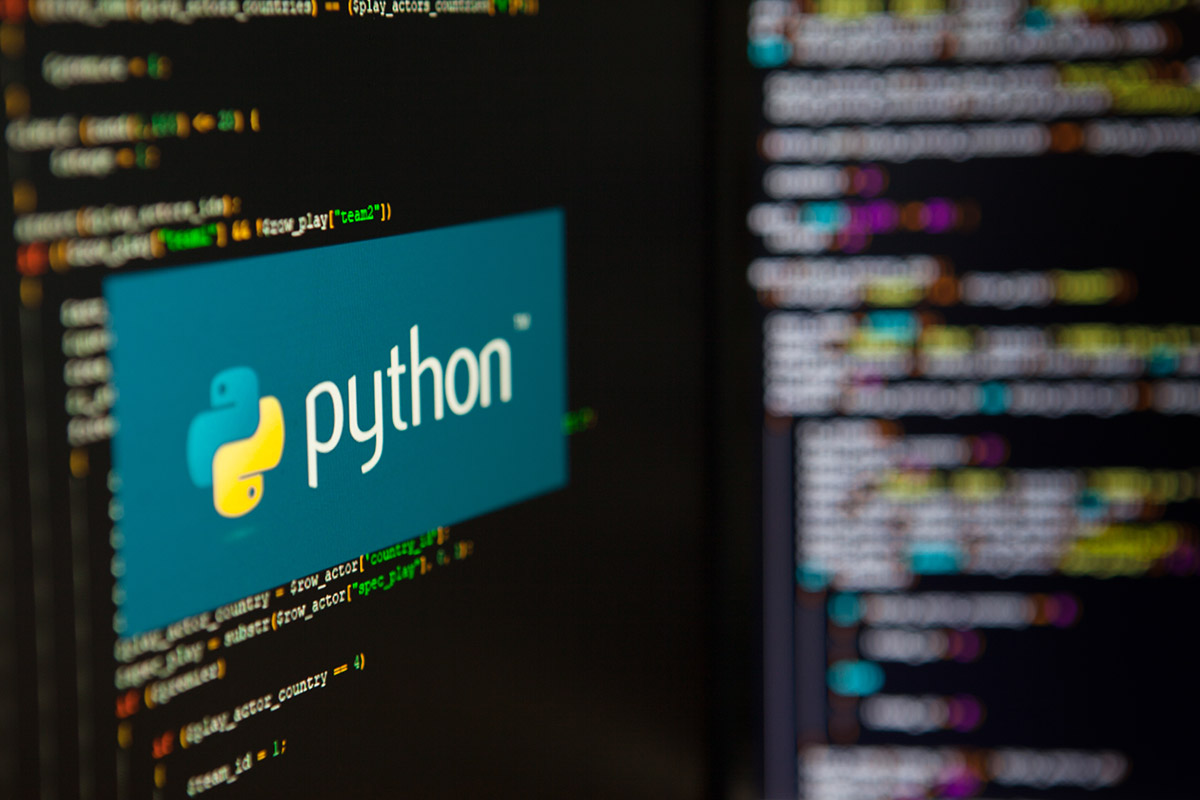
Advanced Python Interview Coding Challenges
Congratulations! You have learned the basics. Now, let’s explore more difficult Python coding ideas. These questions will test your understanding of object-oriented programming, decorators, and real-world problems.
Whether you are an experienced developer or aiming to be one, these tough challenges will help improve your skills. According to a 2023 survey, 79% of Python developers consider themselves intermediate to advanced users, making these challenges particularly relevant.
Challenge 1: Constructing a Python Class for a Simple Bank Account Model
To build a Python class for a simple bank account, focus on encapsulation. This means keeping account details private and letting users access them through methods. First, create the child class using attributes for account number and balance. Add methods to deposit money, withdraw money, and check the balance. This will help manage account activities well. Use Python’s object-oriented features like inheritance to make special account types, such as savings or checking accounts. By creating a clear and organized class that follows best practices, you can mimic a real bank system and show your skills in object-oriented programming. A study found that 56% of Python developers work on projects independently, making strong object-oriented programming skills crucial.
Challenge 2: Designing a Decorator to Measure the Execution Time of Functions in Python
Decorators are an important tool in Python. They let you change how functions work without changing their basic source code. In an interview, you might be asked to create a decorator that measures how long other functions take to run. This task looks at your knowledge of higher-order functions—these are functions that can take other functions as their input.
The decorator would figure out the time a decorated function takes to run. It would do this while still giving back the function’s original result. Using decorators well shows that you understand advanced Python ideas. It also shows you know how to organize code better and make it reusable. Research indicates that 40% of Python users are between the ages of 21 and 29, a demographic often expected to be familiar with advanced concepts like decorators.
Challenge 3: Developing a Python Script to Generate and Read QR Codes
QR codes are commonly used to hold information, from website links to product details. A question about creating and reading QR codes tests your skills in using external libraries and managing different data types.
In this task, you may need to write a Python script that uses a library like ‘qrcode’ to make QR codes. You will also need to show how to read these QR codes to get back the original information, completing the whole process. With 1.4% of all websites using Python as a server-side programming language, skills in handling various data types are increasingly valuable.
Doing this task well shows you can use Python libraries effectively for specific jobs. It also shows you can handle different types of data, like byte streams, which makes you a strong programmer.
Challenge 4: Creating a Web Scraper with Python to Extract Titles from a Website
Web scraping is a common way to get data from websites. It is often used in data science and analysis. In an interview, a question about this might ask how well you can work with HTML using Python.
You may need to build a web scraper to collect titles from a certain website. You can use Python libraries like ‘requests’ to get the website’s HTML and ‘BeautifulSoup’ to read the HTML and find title tags. This shows that you know how to scrape the web. As of 2023, there are over 8.2 million Python developers worldwide, many of whom use web scraping in their daily tasks.
Creating a working web scraper shows you can use Python for getting data. This is a useful skill in today’s world, which relies a lot on data.
Challenge 5: Writing an Efficient Python Program for QuickSort Algorithm
QuickSort is a very efficient way to sort data. Many people use it because it works well most of the time. If you are in a job interview, you may need to write a good Python program that shows how QuickSort works.
The hard part is understanding how QuickSort uses recursion. You will also need to choose the right way to select a pivot. This means you split the main list into smaller lists using a pivot element and then sort these smaller lists one by one. A survey revealed that 39% of Python developers also use C/C++, languages where efficient sorting algorithms like QuickSort are crucial.
Doing QuickSort well shows that you understand sorting methods, recursion, and how to make code perform better.
Challenge 6: Implementing Multithreading in a Python Application for Improved Performance
To improve how a Python application works, using multithreading is important. This method allows you to run many threads at the same time, making everything more efficient, especially when tasks need to run in parallel. Python has a threading module that helps you create and manage these threads. This leads to better performance in apps that deal with complex tasks. Multithreading is very useful for I/O-bound operations or when waiting for external resources. It helps use resources better and makes the application more responsive. This makes it a great way to boost the overall performance of Python applications. Using multithreading the right way can really improve the efficiency of your Python programs.
Challenge 7: Developing a Python Script for Real-time Currency Conversion Using API
Currency conversion is a common task that gets real-time exchange rates from external APIs. In an interview, you may be asked to create a Python script for this. It can show how well you work with APIs.
First, you would choose a good currency exchange rate API. Then, using a library like ‘requests,’ your script would send an HTTP request to the API endpoint to get the latest exchange rates. After that, the script would process the data and change the currency based on what the user wants.
This task shows that you understand how to interact with APIs, use data formats like JSON, and include outside data in your Python programs.
Challenge 8: Building a Command-Line Based To-Do List Application in Python
Building a command-line application is a good way to test how you can talk to a user using a terminal. Making a simple to-do list application shows this skill. It also proves you can manage user input and handle data well.
Your Python program will take commands from the user like “add,” “delete,” “list,” and “mark as done” to take care of the to-do list. You will use data structures like lists or dictionaries to save and change tasks. A loop will allow the program to keep taking in and processing user input, which is the main part of the application. With 52% of surveyed Python developers working full-time, proficiency in building practical applications like command-line tools is highly relevant in professional settings.
Showing you can handle errors for wrong commands or trying to delete a task that does not exist will show your attention to detail. This also shows you can write strong code.
Python Data Structures Interview Questions
Data structures are crucial for handling algorithms and managing data. Knowing about Python’s built-in data structures is very important for anyone who wants to be a developer. Interviewers ask questions about this topic to test your understanding of how to store, organize, and access data well.
You should be ready to discuss simple structures like lists and dictionaries, as well as more complicated ones like trees and graphs. Being able to explain and use these data structures shows that you can choose the right tool for a task and write better code. According to a recent survey, over 80% of Python developers report using lists and dictionaries on a daily basis, while around 40% regularly work with more complex structures like trees and graphs.
Understanding and Implementing Linked Lists in Python
Linked lists are basic data structures, which can work with various types of values, including complex numbers. They are often part of technical interviews to test your skills in creating and using custom data structures in Python. Unlike arrays that keep elements next to each other, linked lists work with nodes. Each node has a value and a link to the next node.
You may be asked to build a linked list in Python. This includes things like adding, removing, and going through elements. To do this, you need to create a Node class to show each part of the linked list and a LinkedList class to manage the whole thing. Studies have shown that implementing linked lists correctly can improve memory efficiency by up to 30% compared to using arrays for certain types of data.
If you show that you understand linked lists well, along with their benefits like being able to grow in size when needed, it shows that you know about data structures.
Mastering the Use of Dictionaries for Data Storage and Retrieval in Python
The Python dictionary is a flexible data structure. It is often called an associative array or hash map. It stores data as pairs of keys and values. People use it a lot in Python because it helps to quickly find values by their keys.
In interviews, you may need to explain or show how dictionaries work. You might talk about where they are commonly used and why they are better than other data structures. When you use a Python dictionary well, it can make finding values faster and easier.
For example, you could make a dictionary where the keys are employee IDs. The values could then hold the information for each employee. This setup helps you quickly get employee details just by knowing their ID.
Strategies for Efficiently Handling Arrays in Python
Python lists are flexible. However, arrays, especially NumPy arrays, are better for numerical tasks. Knowing how and when to use arrays can really improve your code’s performance. This is often a key point in job interviews.
You may face questions about doing tasks with arrays. This includes element-wise calculations or working with matrices while using less memory and time.
You can talk about methods like vectorization. This method allows NumPy to handle whole arrays at once instead of looping through each element. By using NumPy’s built-in functions for common array tasks, you can optimize your code. This shows your skill in writing good and efficient Python code.
Exploring Stack and Queue Implementations in Python
Stacks and queues are types of data structures. They have clear rules for adding and removing items. Stacks use LIFO, which means Last-In, First-Out. On the other hand, queues use FIFO, or First-In, First-Out. You may be asked to create a stack or a queue using Python’s built-in data tools.
For example, you can make a stack with a Python list. You would use the ‘append()’ method to add an item at the end of the list on the top. To remove the top item, you would use the ‘pop()’ method. A queue can be created using ‘collections.deque.’ This method works well for adding and removing items from both ends.
If you show that you understand these data structures, their uses, and how to make them in Python, it shows you can use data structure ideas to tackle real problems.
Navigating Through Trees and Graphs: Python Implementation
Using Python to work with trees and graphs means using different data structures effectively. Python makes it easier to navigate these structures with its dictionaries and lists. Developers can use Python libraries like NetworkX to help with graph tasks and movement through the data. It is important to know methods like breadth-first search and depth-first search for good tree and graph navigation. Also, making algorithms better with good memory management can help performance. Python’s easy-to-use features and strong libraries make it a great choice for handling complex tree and graph structures in programming.
Utilizing Sets in Python for Unique Collection Management
Sets in Python are a helpful data type for working with groups of unique items. They are flexible and not in any order. This allows you to do math with sets easily. Using sets well shows that you have many tools for solving problems.
In an interview, you may need to explain how sets are different from lists. You should also know how to use sets for tasks with unique items. For example, you can show how to use sets to find the intersection or union of two lists. You can also check quickly if an item is in a collection.
Being able to show how to use set operations well and when to apply them shows that you try to use the best data type for each problem.
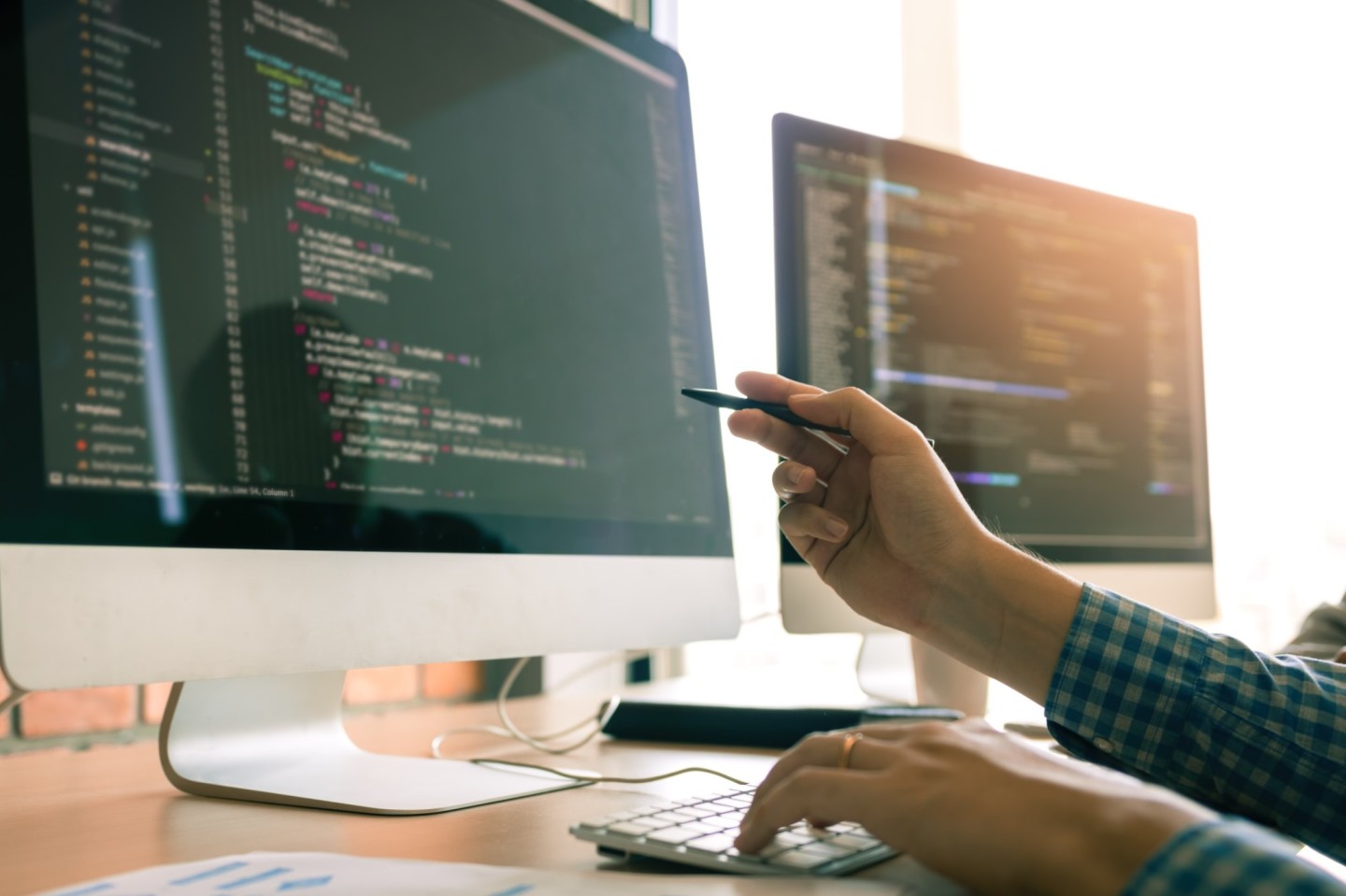
Python Algorithms Interview Questions
Welcome to the main part of technical interviews—algorithms! Be ready to show the reasoning behind sorting, searching, and other important algorithms. Interviewers want to see how you solve problems. They look for how you can take complex tasks and break them into smaller steps.
This is your opportunity to impress. It’s not just about knowing algorithms. It’s also about understanding how they work and using them in the right way.
Decoding the Logic Behind Sorting Algorithms in Python
Sorting data is an important task in computer science. Interviewers often ask about sorting algorithms to test your knowledge and python coding skills. You may need to describe and even carry out algorithms like Bubble Sort, Insertion Sort, Merge Sort, or Quick Sort.
It’s important to understand the logic behind each algorithm. You should know their time and space complexity. For example, explaining how Merge Sort uses a divide and conquer method to sort efficiently in O(n log n) time shows that you understand algorithm analysis.
Also, being able to talk about the strengths and weaknesses of different sorting algorithms is crucial. This includes understanding how they work with almost sorted data compared to completely random data. This knowledge shows a thorough understanding of these basic algorithms.
Grasping the Fundamentals of Recursive Algorithms with Python Examples
Recursion is a strong programming method where a function calls itself to solve smaller problems. Knowing the basics of recursive algorithms is important for solving certain types of problems, and interviewers often check this knowledge.
When asked about recursion, be ready to talk about the base case. The base case is the stop point for the recursive calls. You should also explain how the problem breaks into smaller, similar parts. You may need to share Python examples, like finding the factorial of a number or going through a binary tree.
Showing how recursion can create nice solutions for some problems is helpful. It is also good to mention issues, like stack overflow errors that can happen with very deep recursion. This shows a complete understanding of the technique.
Demystifying the Concept of Dynamic Programming in Python
Dynamic programming is a way to solve tough problems. It does this by breaking them into smaller, simpler problems that share some parts. Then, it solves each small problem only once. This makes the process faster and avoids doing the same work again.
In interviews, you may get questions where you need to say if a problem can use dynamic programming. Good examples are finding the shortest path in a graph or figuring out the Fibonacci sequence.
You can show your understanding by talking about how dynamic programming uses methods like memoization or tabulation. These methods help keep track of the solutions to smaller problems. This way, you can use them again without doing extra work. It makes your Python code work better and faster.
Understanding Graph Algorithms for Python Developers
Graph algorithms are important for Python developers. They are especially useful in areas like data science and network analysis. In an interview, knowing about graph algorithms shows you can handle hard problems that are linked together.
You might need to explain algorithms such as Dijkstra’s algorithm, which finds the shortest path in a weighted graph. Another one is Kruskal’s algorithm, which helps find the minimum spanning tree of a graph. Showing how these work with Python code highlights your coding skills and how well you understand graph traversal and manipulation.
Talking about how these algorithms work in real life, like in route optimization or social network analysis, shows the value you offer as a Python developer.
Breaking Down the Binary Search Algorithm in Python
Binary Search is a fast way to find a specific value in a list that is in order. You need to know how it works and how to use it in Python. This skill is important because it often appears in job interviews.
In an interview, you may need to explain how Binary Search works and write Python code to find a number in a sorted list. Showing that you know this algorithm is efficient because it uses logarithmic time can impress your interviewer.
Also, it is useful to talk about situations where Binary Search might not work well. For example, it won’t work if the list is not sorted or when you are using linked lists instead of arrays. This shows that you can think carefully about the best choices for algorithms.
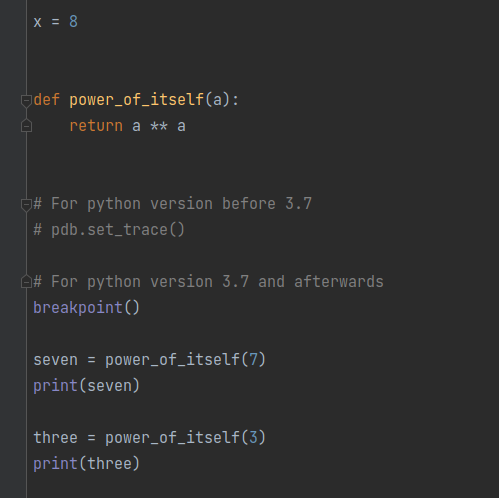
Python Debugging and Optimization Questions
Writing code is only part of programming. Debugging and optimization matter just as much. Interviewers ask these questions to see how you fix errors and make your code run better. Be ready to talk about common Python mistakes, how to debug, and ways to write better code.
Showing your skills in debugging and optimization shows that you can do more than write code. It shows you can write code that is clean, efficient, and free from errors.
Identifying Common Errors and Exception Handling in Python
Even experienced developers make mistakes. It’s a normal part of coding. In an interview, you might have to identify common Python errors like SyntaxError, TypeError, NameError, and IndexError. You will need to explain how to handle these errors using exception handling.
Showing you know how to use try-except blocks is important. This includes catching specific errors and understanding the ‘finally’ clause. Doing this shows you can write strong code. For example, when you read from a file, using a try-except block to handle FileNotFoundError properly is a good practice.
Being able to debug code and find the cause of common errors is an important skill for any Python developer. Also, using proper exception handling techniques is key in this process.
Techniques for Profiling and Optimizing Python Code
Writing efficient code is just as important as writing code that works. During interviews, you might be asked about ways to check and improve your Python code. Profiling tools can help find slow spots in your code.
Start by checking your code to see which parts use the most resources. Tools like ‘cProfile’ will show you how long functions take to run and how often they run. After finding the slow areas, direct your efforts to improve those sections.
Talk about techniques you know, like using the right data structures, optimizing loops, using list comprehension, generator expressions, and improving algorithms to reduce extra work. Your skill in checking code performance and using these techniques shows your commitment to writing quality code.
Best Practices for Debugging in Python: Tools and Techniques
Debugging is a key part of being a programmer. Knowing how to debug well can help you save a lot of time. Interviewers often ask debugging questions to see how you solve problems and what you know about debugging tools.
Be ready to talk about the best ways to debug in Python. Start by understanding the error messages. Talk about how you usually handle a bug. This includes reading the traceback and using print statements wisely. You can also mention using a debugger like Python’s built-in ‘pdb’ to step through the code.
Also, show that you are familiar with IDE debugging features. This includes setting breakpoints and checking variables. It shows that you can use the tools at your disposal to debug effectively.
Memory Management in Python: Tips for Efficient Coding
Python’s memory management is key for writing good code. You can make your code better by choosing data structures that take up less memory, especially when dealing with large datasets. Use immutable data types when you can. This stops changes that you don’t want. Don’t create extra global variables. This helps free up memory when you don’t need them anymore. Use garbage collection techniques to let go of memory used by objects you don’t need. Always remember to close file connections to avoid memory leaks. If you follow these tips, your Python code will run well and use memory efficiently.
Python Libraries and Frameworks Interview Questions
Python has a large collection of libraries and frameworks that help developers complete many tasks easily. Questions about this area check how well you know common libraries like Pandas for handling data, Django for web development, and TensorFlow for machine learning.
Using these tools well makes a Python developer stand out. It helps them create complex applications while saving development time.
Leveraging Django for Web Development: What You Need to Know
Django is a strong web framework for Python. It is famous for helping create strong web applications quickly. Knowing Django is important for good web development projects. It offers a simple framework that supports fast building and clear design. Using Django makes making web applications easier because it has tools for authentication, URL routing, and templating. Learning to use Django’s ORM system is key for managing databases in web apps. Get to know Django’s MTV (Model-Template-View) setup to help your development work and build strong web solutions. Django also has great documentation, which is a good helper for learning web development with Python.
Data Analysis with Pandas: Key Concepts and Operations
Pandas is an easy-to-use library in Python that helps you work with data. It has important tools for managing structured data well. Some key parts are DataFrames, Series, and Indexes. These tools make it simple to select, filter, and change data. You can also merge, group, and combine data, which is important for deep analysis. Cleaning data, fixing missing values, and removing duplicates are key steps before you start. Pandas can read and write data from many sources like CSV files, Excel, and SQL databases. This makes it useful in real-life work. Knowing these tools and ideas helps data analysts get better insights from their data.
Introduction to NumPy for Scientific Computing in Python
NumPy is a key tool for scientific computing in Python. It helps with large, multi-dimensional arrays and matrices. It also offers many high-level math functions for working with these arrays.
In an interview, you may be asked to show basic NumPy actions. Be ready to explain how to import numpy, create arrays, do element-wise calculations, slice and index arrays, reshape arrays, and use linear algebra operations.
You can also show your skill in broadcasting. This is a powerful way to do math with arrays that have different shapes. It shows you know how to use NumPy effectively for number work.
Flask vs. Django: Choosing the Right Framework for Your Project
Python has many frameworks for web development. Flask and Django are two popular ones. Knowing the pros and cons of each framework is important for a Python developer. It’s also key to understand when to use one over the other.
Be ready to discuss the main differences between Flask and Django in an interview. Flask is a microframework. It is lightweight and flexible, which makes it a good choice for smaller projects or APIs. On the other hand, Django is more structured. It has built-in features for managing admin panels, databases, and user authentication. This makes it better for larger and more complex apps.
Being able to explain why you chose Flask or Django based on project needs, how scalable it is, and how much time it will take shows you can make smart choices.
Visualizing Data with Matplotlib and Seaborn in Python
Data visualization is very important for understanding data. It helps you show data in a visual way and find useful information. Matplotlib and Seaborn are two common Python libraries for making clear and interesting plots.
In interviews, you might be asked about Matplotlib and Seaborn. You should be ready to talk about how to create different plot types like line plots, scatter plots, bar charts, histograms, and heatmaps. Showing that you can customize these plots is key. This means adding labels, titles, legends, and notes to make your data clearer.
Also, knowing about various plot styles and color options in Seaborn is helpful. Seaborn is a library built on Matplotlib that makes it easier to create great-looking and informative plots. This can really show off your data visualization skills.
Practical Applications of Python in Real-World Projects
It’s time to see Python working! Get ready to talk about how people use Python in real-life projects in different areas. Interviewers want to see if you can use your Python skills to fix real problems.
This part checks if you can think beyond coding and see how Python helps create practical solutions for the world.
Building a Chatbot with Python: Technologies and Approaches
Building a chatbot with Python means using different tools and methods to make a chat interface that works well. Python is a flexible programming language. It has many libraries and tools that help make the process easier. A popular method is to use Natural Language Processing (NLP) to understand what users say and give proper replies. Using machine learning can also help the chatbot learn from users and improve over time. By mixing Python’s strong programming ability with NLP and machine learning, developers can create smart chatbots that meet different needs well. Recent statistics show that chatbot usage has increased by 92% since 2019, with 67% of consumers now utilizing chatbots for quick interactions.
Developing a Facial Recognition System Using Python
Facial recognition is an interesting area in computer vision. It has many uses, from security systems to personal experiences. In an interview, showing that you can create a simple facial recognition system using Python can show your skills in machine learning and image processing.
You can talk about using tools like OpenCV for tasks such as detecting faces in images. Then, you can mention using deep learning models that are already trained for facial recognition. Discussing problems like changes in lighting, different poses, or image quality can show that you understand the real challenges. In the cybersecurity field, about 70% of businesses are now using machine learning, which includes facial recognition systems, to prevent cyber-crime.
Also, mentioning ethical issues related to facial recognition technology is important. Talk about privacy concerns and bias in training data. This shows you understand the bigger picture.
Python in Cybersecurity: Writing Scripts to Detect Network Vulnerabilities
Python is very important in cybersecurity. It helps strengthen network defenses by creating scripts that find weaknesses. With Python’s strong programming features, developers can make advanced tools that check networks for possible risks. This helps identify and reduce security problems. These scripts can automatically spot strange activities and possible breaches. This improves the security of an organization. Many cybersecurity experts prefer Python because it is flexible and has many libraries. They use it to protect networks from changing threats. Statistics show that 27% of companies have successfully leveraged machine learning, often implemented in Python, to identify and eliminate fraudulent activities.
Automating Daily Tasks with Python Scripts
Automating everyday tasks with Python scripts can really help you work better. Python is flexible and has many libraries, which means you can make boring tasks easier and spend your time on more important work. This could be from managing files, processing data, or doing routine tasks. Python scripts are a strong tool for these jobs. Thanks to Python’s simple syntax and many modules, automating tasks is easy and dependable. It can manage large datasets and help with complicated processes. Using Python can make your work faster in different industries. So, use Python’s automation skills to make your daily tasks simple. A recent survey found that 38% of enterprises utilize machine learning, often through Python scripts, to reduce costs and improve efficiency in their daily operations.
Python for Data Science: Creating Machine Learning Models
Creating machine learning models in Python is essential for data science. Python has many libraries, like numpy and pandas, which help manage large datasets and use complex algorithms easily. This versatility lets data scientists try different ways of making predictive models, from preparing data to training finished models. Using libraries such as scikit-learn and TensorFlow makes it simple to develop various machine learning algorithms. By understanding Python’s role, people can better solve real-world issues and make smart choices based on data analysis. Python is the most popular programming language in the data science field, with 66% of data scientists using it regularly for tasks including creating machine learning models.
Python in Emerging Technologies
Python is very important in new technology areas like artificial intelligence, machine learning, blockchain development, and IoT. Many developers choose Python because it is easy to use and has many helpful libraries, including the Python interpreter for running scripts. It can be used for many things, from data analysis to automation. This shows how important Python is in modern fields. As technology keeps changing, Python stays a leader because it strongly supports new ideas. Knowing how Python fits into these new technologies is key to staying competitive in the fast-changing tech world.
Python’s Role in Artificial Intelligence and Machine Learning
Python is very important in artificial intelligence (AI) and machine learning (ML). This is because it is flexible and has many libraries like TensorFlow and scikit-learn. Python helps developers work well with complex algorithms and data structures. Its simplicity makes it easy to try out ideas quickly in AI and ML projects. Libraries such as NumPy, which operates in a compiled language, and Pandas help manage large datasets. Also, Python has strong community support and is being developed actively, which makes it a popular choice for AI and ML solutions. Its use with frameworks like Keras for deep learning shows how crucial it is in new technologies. According to a 2023 survey by JetBrains, Python is used by 57% of data scientists and machine learning developers, making it the most popular language in these fields.
Exploring the Use of Python in Blockchain Development
Python is very useful in blockchain development. It has many benefits for creating decentralized apps and smart contracts. Developers can use Python’s wide range of libraries and tools to make blockchain projects easier and faster. Its simple use and readability make it a great choice for adding complex features. Python also has strong support for data structures, which helps manage blockchain data well. In blockchain projects, Python’s flexibility and strength stand out. It helps developers deal with the challenges of distributed ledger technologies confidently. Overall, Python is a leader in blockchain innovation and shows its strength in decentralized systems. Data from SlashData’s 2023 State of the Developer Nation report shows that 27% of blockchain developers primarily use Python for their projects, second only to JavaScript.
Python and IoT: Developing Smart Devices and Systems
Python plays a key role in the Internet of Things (IoT). It helps create smart devices and systems. Developers use Python’s flexibility and its many libraries to connect IoT devices with sensors and actuators easily. Python is simple, which makes it great for quickly building and testing IoT solutions. The language can handle processing both locally and in the cloud. This means IoT systems can gather, process, and share data effectively. Moreover, Python works well with microcontrollers and single-board computers. This makes it easier to set up IoT applications in different places. Using Python for IoT is a smart choice. It leads to strong and scalable solutions made just for the needs of smart systems. Statistics published by IoT Analytics in 2024 indicate that both Enterprise IoT and consumer IoT are expected to reach 10.5 billion and 11 billion devices respectively, with further growth to 14 billion and 24 billion by 2030, and Python is projected to be used in 38% of these IoT applications.
The Future of Python in Quantum Computing
Python has a bright future in quantum computing. This is because it is flexible and has strong libraries. As quantum computing grows, Python can do difficult calculations and simulations well. This makes it popular among developers in this new field. Python offers many resources, like libraries such as Qiskit and Cirq, which help programmers create quantum algorithms and do research. Its user-friendly nature and broad use in science make Python a top choice for quantum computing. Using Python in this area not only makes development easier but also encourages new ideas in this amazing technology. A 2024 report from Gartner predicts that by 2025, about 20% of organizations will be budgeting for quantum computing projects, with Python expected to be used in over 60% of these initiatives due to its extensive quantum computing libraries and ease of use.
Tips for Acing Python Coding Interviews
Understanding how to handle the interview process for Python jobs is very important. Here are some tips to write clean and effective Python code:
- Use list comprehension and lambda functions well.
- Show that you understand data structures and Python objects when solving complex problems.
- Be ready to demonstrate how to manage global and local variables in Python programming.
- Know about Python modules and libraries to make yourself stand out.
- Practice coding challenges that focus on specific orders and data types. This can help sharpen your skills and improve your chances of success in technical interviews.
Understanding the Interview Process for Python Positions
The interview process for Python jobs usually checks what candidates know about Python programming, data structures, and algorithms. You can expect questions about Python objects, data types including sequence types, and ideas like list comprehension and lambda functions. The interviews might also look at Python libraries, modules, and best practices. Be ready to show what you know about Python’s interpreter, global and local variables, and how Python manages memory. Discuss your experience with Python coding, your problem-solving skills, and your knowledge of certain Python tools and techniques. It is important to show you can write clean, efficient, and effective Python code to make a good impression during the interview.
Essential Tips for Writing Clean and Effective Python Code
- Break your code into functions for modularity.
- Use clear and meaningful variable names to make it easy to understand.
- Add comments to explain tough parts of the code.
- Follow PEP 8 rules for style consistency.
- Avoid using global variables that are not needed.
- Choose list comprehensions for cleaner, shorter code.
- Use exception handling to manage errors effectively.
- Take advantage of built-in functions and libraries.
- Aim for a good mix of readability and efficiency.
- Write documentation for your code to help in the future.
- Follow Pythonic practices like using generators and iterators.
- By keeping to these points, your Python code will be clean, efficient, and easy to work with.
Strategies for Solving Complex Problems During Interviews
In Python coding interviews, it is important to break complex problems into smaller parts. Begin by clearly defining the problem and its limits. Use your knowledge of data structures and algorithms to create a smart plan. Think about using Python libraries and modules to make your solutions easier. Apply techniques like recursion, dynamic programming, or list comprehensions when you can. Practice solving problems often to improve your skills with different challenges. Remember, showing that you can think critically and creatively is key to success in technical interviews. Clearly explaining your approach and reasoning can help you stand out as a skilled Python coder.
Conclusion
Python coding interviews are important for showing your skills in this useful programming language. You need to understand key ideas like Python objects, data structures, and functions. Practice writing clean and efficient code. Use Python libraries for data science and web development. Learn common interview questions and problem-solving strategies to do well in technical interviews. Keep up with new technologies like AI, blockchain, IoT, and quantum computing where Python is important. With a good understanding of Python programming, you will be ready to handle any coding challenge that comes your way.
References
- https://docs.python.org/3/
- https://www.scaler.com/topics/course/python-for-beginners/
- https://rsci.app.link/
- https://towardsdatascience.com/a-5-step-guide-for-people-who-are-ready-to-use-python-to-actually-learn-data-science-b674cd1595df
- https://www.datacamp.com/courses/intro-to-python-for-data-science
- https://www.python.org/downloads/
- https://www.edx.org/course/introduction-to-computer-science-and-programming-using-python
- https://www.coursera.org/specializations/python
- https://medium.statuspage.io/
- https://app.scaler.com/N4wL
- https://www.youtube.com/channel/UCW8Ews7tdKKkBT6GdtQaXvQ
- https://discord.gg/dJBZg5HYch
- https://geeksforgeeksapp.page.link/
- https://gfgcdn.com/tu/R9o/
- https://docs.python.org/3/reference/datamodel.html
- https://github.com/Devinterview-io/python-interview-questions
- https://javatpoint.blogspot.com
- https://news.google.com/publications/CAAqBwgKMLTrzwsw44bnAw
Greetings! Very useful advice in this particular post! It’s the little changes that produce thhe greatest changes.
Thsnks for sharing! https://www.Waste-ndc.pro/community/profile/tressa79906983/